Java Programming A Complete Guide for Aspiring Developers
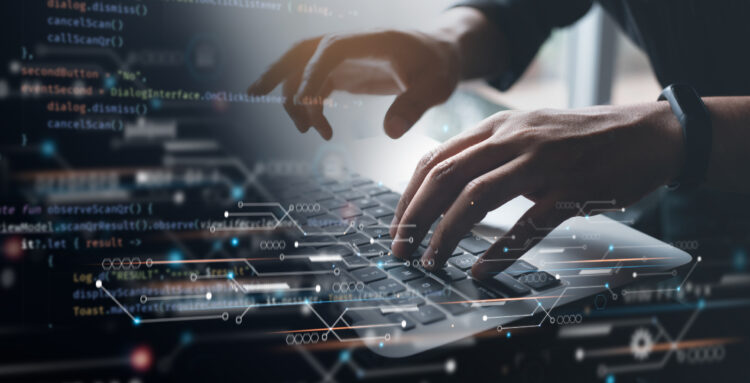
By
Java, one of the most widely used programming languages in the world, has cemented its place as a cornerstone in the software development industry. With its platform independence, security features, and vast ecosystem, Java offers endless opportunities for developers across various domains, from web development and mobile applications to enterprise-level systems and beyond. If you’re an aspiring developer eager to learn Java, this comprehensive guide is crafted to help you navigate your learning journey from a beginner to a proficient coder.
Why Learn Java?
Java is renowned for its versatility, reliability, and scalability, making it a preferred choice for many developers and organizations worldwide. Developed in the mid-1990s by Sun Microsystems (now owned by Oracle Corporation), Java has grown into a mature language that powers a wide array of applications, from Android mobile apps and large-scale enterprise systems to complex scientific applications.
One of the key reasons to learn Java is its “write once, run anywhere” philosophy. Java code, when compiled, can run on any device that has a Java Virtual Machine (JVM), regardless of the underlying hardware or operating system. This platform independence is a significant advantage in today’s diverse computing environment, where applications need to function across multiple platforms.
Moreover, Java’s strong memory management, robust security features, and extensive libraries and frameworks make it an ideal language for developing scalable and secure applications. Learning Java not only opens up numerous job opportunities but also provides a strong foundation in programming, making it easier to learn other languages like C++, Python, or JavaScript in the future.
Getting Started: Understanding the Basics
For beginners, the first step in learning Java is to get familiar with its syntax and basic concepts. Java’s syntax is relatively straightforward and similar to other C-based languages, which makes it easier for those with some programming experience to pick up. However, even if you’re new to programming, Java is an excellent starting point due to its clear and consistent syntax.
Begin by understanding fundamental concepts such as variables, data types, and operators. Java’s type system is static, meaning you need to define the data type of a variable before using it. This might seem strict, but it helps in catching errors early during the compilation phase, making your code more reliable.
Control structures such as loops (for, while) and conditionals (if, switch) are essential for making decisions in your programs and controlling the flow of execution. Practice by writing simple programs, such as calculating the sum of an array of numbers or determining if a number is prime.
Java is also an object-oriented programming (OOP) language, which means it is based on the concept of “objects” that represent real-world entities. OOP is a powerful paradigm that allows for modular, reusable, and maintainable code. To start, you should familiarize yourself with basic OOP concepts like classes, objects, methods, and constructors. Understanding these concepts will set the foundation for more advanced topics like inheritance, polymorphism, and encapsulation.
Setting up your development environment is another crucial step. You’ll need to install the Java Development Kit (JDK), which includes the necessary tools for compiling and running Java programs. Additionally, using an Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or NetBeans can significantly enhance your productivity by providing features like code completion, debugging, and project management.
Deep Dive into Core Java Concepts
Once you have a solid grasp of the basics, it’s time to dive deeper into core Java concepts. Understanding these will help you build more complex applications and give you a better grasp of how Java manages data and processes.
Exception Handling is a crucial aspect of writing robust Java programs. It allows your program to handle unexpected events or errors gracefully without crashing. By using try-catch blocks, you can catch exceptions and take appropriate actions, such as logging the error or providing a user-friendly message.
The Collections Framework is another vital area to explore. Collections are data structures that store and manage groups of objects. The Java Collections Framework provides various interfaces (List, Set, Map) and their implementations (ArrayList, HashSet, HashMap) that help in storing, retrieving, and manipulating data efficiently. Understanding how to use collections effectively is key to managing data in your applications.
Input/Output (I/O) Streams in Java are used for reading from and writing to data sources like files, network connections, or memory buffers. Java provides a comprehensive set of I/O classes in the java.io package that allow you to perform tasks like file reading/writing, buffering, and data serialization. Mastering I/O streams is essential for applications that need to interact with external data sources.
Multi-threading is another advanced topic that enables your Java programs to perform multiple tasks simultaneously. Java provides built-in support for multi-threading with the java.lang.Thread class and the java.util.concurrent package. By understanding thread synchronization, you can ensure that your multi-threaded programs run smoothly without issues like race conditions or deadlocks.
Object-Oriented Programming (OOP) in Java
As mentioned earlier, Java is an object-oriented language, and mastering OOP principles is crucial for developing modular, maintainable, and reusable code. Let’s dive deeper into the core OOP concepts in Java:
Encapsulation involves bundling the data (attributes) and the methods that operate on the data into a single unit or class. By using access modifiers like private, protected, and public, you can control the visibility of the class members and protect the data from unintended access.
Inheritance allows one class to inherit the properties and behaviors of another class. This promotes code reuse and establishes a hierarchical relationship between classes. For instance, a subclass can extend a superclass and add additional functionalities while retaining the base characteristics.
Polymorphism enables objects of different classes to be treated as objects of a common superclass. The most common use of polymorphism in Java is method overriding, where a subclass provides a specific implementation of a method already defined in its superclass. This allows for dynamic method invocation, making your code more flexible and scalable.
Practice these OOP principles by working on small projects like an inventory management system, where you can create classes for products, categories, and suppliers, and use inheritance and polymorphism to extend functionalities.
Advanced Topics: Beyond the Basics
As you continue your Java journey, you’ll encounter more advanced topics that will allow you to write more efficient, concise, and scalable code.
Java Generics enable you to write type-safe code that works with any data type. Generics allow you to create classes, interfaces, and methods that can operate on objects of various types while providing compile-time type safety. For example, you can create a generic class for a collection that can hold any type of object without needing to specify the exact type upfront.
Lambda Expressions and the Streams API are features introduced in Java 8 that bring functional programming concepts to Java. Lambda expressions allow you to write anonymous methods in a more concise way, making your code more readable and reducing boilerplate. The Streams API provides a powerful tool for processing collections of data in a functional style, supporting operations like filtering, mapping, and reducing.
Concurrency is another advanced topic that deals with the simultaneous execution of tasks. Java’s concurrency utilities, such as Executors, Locks, and Atomic variables, help in managing multi-threaded applications more efficiently. Understanding concurrency is crucial for building responsive and scalable applications, especially in a multi-core processing environment.
Design Patterns are tried-and-tested solutions to common software design problems. Patterns like Singleton, Factory, and Observer help in writing code that is more flexible, maintainable, and easier to understand. Familiarizing yourself with these patterns will enhance your ability to design robust applications.
Practical Application: Building Real-World Projects
The best way to solidify your Java skills is by applying what you’ve learned to real-world projects. Start with simple applications like a to-do list, calculator, or a basic web app to get comfortable with writing and organizing code. As you gain confidence, take on more complex projects like a content management system (CMS), an e-commerce site, or a social networking application.
Working on these projects will help you understand the full software development lifecycle, from gathering requirements and designing the architecture to coding, testing, and deployment. Additionally, building a portfolio of projects will be invaluable when it comes to showcasing your skills to potential employers.
Learning Resources and Communities
There are countless resources available to help you learn Java. Online platforms like Codecademy, Coursera, and Udemy offer structured courses that cater to various skill levels. Books such as “Head First Java” by Kathy Sierra and Bert Bates, “Effective Java” by Joshua Bloch, and “Java: The Complete Reference” by Herbert Schildt are excellent resources for in-depth learning.
Joining online communities like Stack Overflow, GitHub, and Java forums can also be incredibly valuable. These platforms allow you to ask questions, share knowledge, and stay updated on the latest trends in Java development. Engaging with the community is a great way to learn from others’ experiences and to find support when you encounter challenges.
Preparing for a Java Career
If you aim to pursue a career as a Java developer, preparation is key. Many technical interviews for Java positions include coding challenges that test your understanding of data structures, algorithms, and problem-solving skills. Websites like LeetCode, HackerRank, and Codeforces provide a vast collection of coding problems to practice.
Beyond coding challenges, understanding common design patterns, database management, and version control systems like Git will give you an edge in the job market. Additionally, gaining experience with popular Java frameworks like Spring and Hibernate can make you more attractive to employers, as these are widely used in enterprise development.
Conclusion Java programming is a rewarding skill that opens doors to various career paths in the tech industry. By following this comprehensive guide, you can systematically build your knowledge and confidence as a Java developer. Remember, consistency is key-practice regularly, work on real projects, and engage with the Java community to continuously improve your skills. For those looking to advance their learning, consider enrolling in a Java training course in Greater Noida to gain hands-on experience and expert guidance. With dedication and effort, you’ll be well on your way to becoming a proficient Java developer, ready to tackle complex challenges and create innovative solutions.
sooperarticles.com
No Comment